11.1 Computational thinking
An algorithm is a sequence of steps that, when followed, leads to the solution of a problem. It has a defined set of inputs and delivers an output. Each step in the algorithm leads to another step or completes the algorithm.
Through this module, you will explore the purposes of algorithms as well as think about the design, analysis and implementation of your own algorithms.
Finding solutions to problems
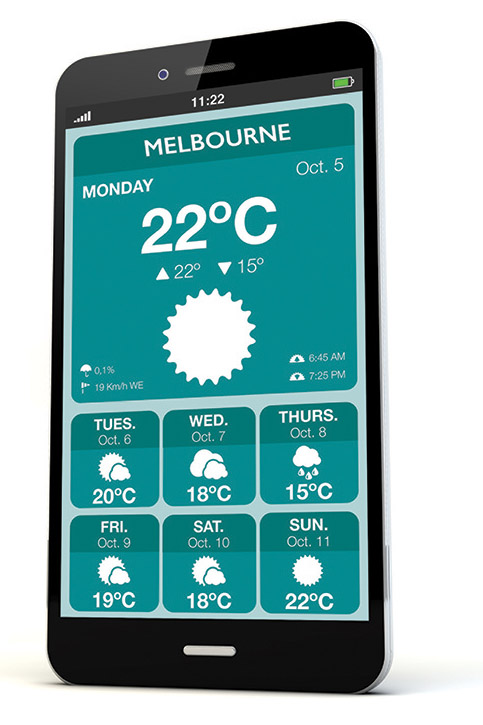
How do people work through complex real-world problems in a systematic way? For example, thousands of apps are available now for smartphones and other digital devices. The detail and extent of functions and features varies enormously. The cost of apps and programs depends on how many people will use them and how much work goes into creating them. But where does the work all start? It begins with the recognition of a problem or requirement. For example, a simple and often used app is the Weather app on a smartphone. The requirement – what is the weather forecast for today for a specific location. The solution – an algorithm that lists the steps to get the desired result – display the weather forecast for today for the location specified.
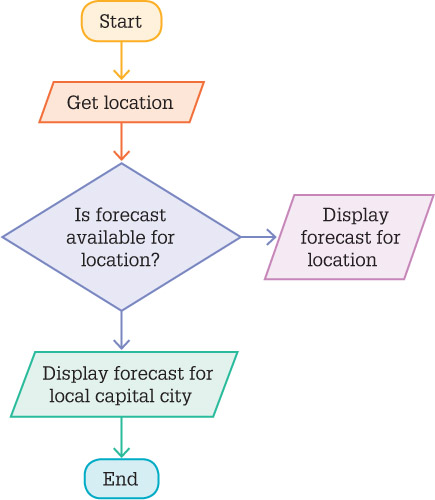
Algorithms help people, such as app developers or computer programmers, decompose or break down a complex problem into smaller, more manageable tasks.
For example, the following algorithm for a weather app is written in point form:
- What is the location for the weather forecast?
- Get forecast for that location.
- Display weather forecast for location, otherwise display local capital city.
This gives us a very simple algorithm.
To work towards creating the program that will solve the problem of how to display a weather app on a smartphone, the app developer or computer programmer will often create a flowchart to visualise the algorithm.
In the flowchart opposite, the diamond shape is a DECISION that creates branching. Branching results when a choice is made between one or more actions depending on the data provided and other conditions.
From this diagram a structured English version can be written that a coder will use to write the code for the software, using language such as conditional IF statements.
Keywords such as START, END, IF, UNTIL, ELSE and THEN are used to provide a type of language (also known as syntax) to assist in identifying the logical steps to describe the algorithm.
Using structured English, the above flowchart would look like this:
START
Get location
IF forecast is available for location
THEN
Get forecast for location and display
ELSE
Get forecast for local capital city and display
ENDIF
END
Working out and displaying the charge for parking is another example of a simple algorithm.
- What is the number of hours a customer has parked in the parking lot?
- If the number is less than 2, parking is free.
- If the parking is more than 5, the maximum fee of $5 is to be charged.
- If the number is more than 2 hours and less than 5, the fee is $1 times the number of hours.
The flowchart would look like this:
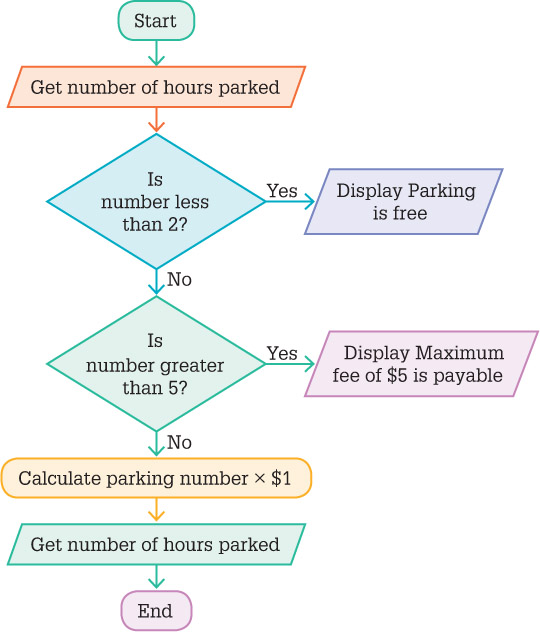
The structured English would look like this:
START
Get number of hours parked
IF number less than 2
THEN
Display “Parking charge is “Free””
ELSE
IF number greater than 5
THEN
Display “Maximum Parking fee of $5 is payable”
ELSE
Calculate parking fee result
By multiplying number by $1
Display the parking fee payable
ENDIF
ENDIF
END
Once an algorithm has been written in steps or drawn on a flowchart it must be tested (through a process known as desk checking) to ensure it is working as expected. You should work through the algorithm with various input data to check this. The data should include both expected and unexpected values. For example, work through the flowchart with data where hours parked are the number 0, 1, 2, 3, 4, 6 or 10.
Algorithms and programming exercise 1
Spreadsheets and testing algorithms
Skills practised
- Understanding algorithms
- Applying algorithmic thinking
Numerical methods using tables, spreadsheets, graphs and other algorithms can be used to solve a range of mathematical equations and to compute other values. For example, a spreadsheet is a calculating program that can be used to implement simple addition algorithms. However, an algorithm like the car parking example can also be used to determine an appropriate formula to solve a problem using a spreadsheet application.
For example, see the exercises in Module 7: Spreadsheets that determine the charge for internet usage. Exercises 10 and 11 use the IF function, which, like an IF statement in an algorithm, give us a range of outputs depending on the various inputs.
To test yourself, write out the algorithm for calculating the internet charge per hour in simple numbered steps. Use the input data provided in Exercise 12, Module 7.
Algorithms outside the classroom
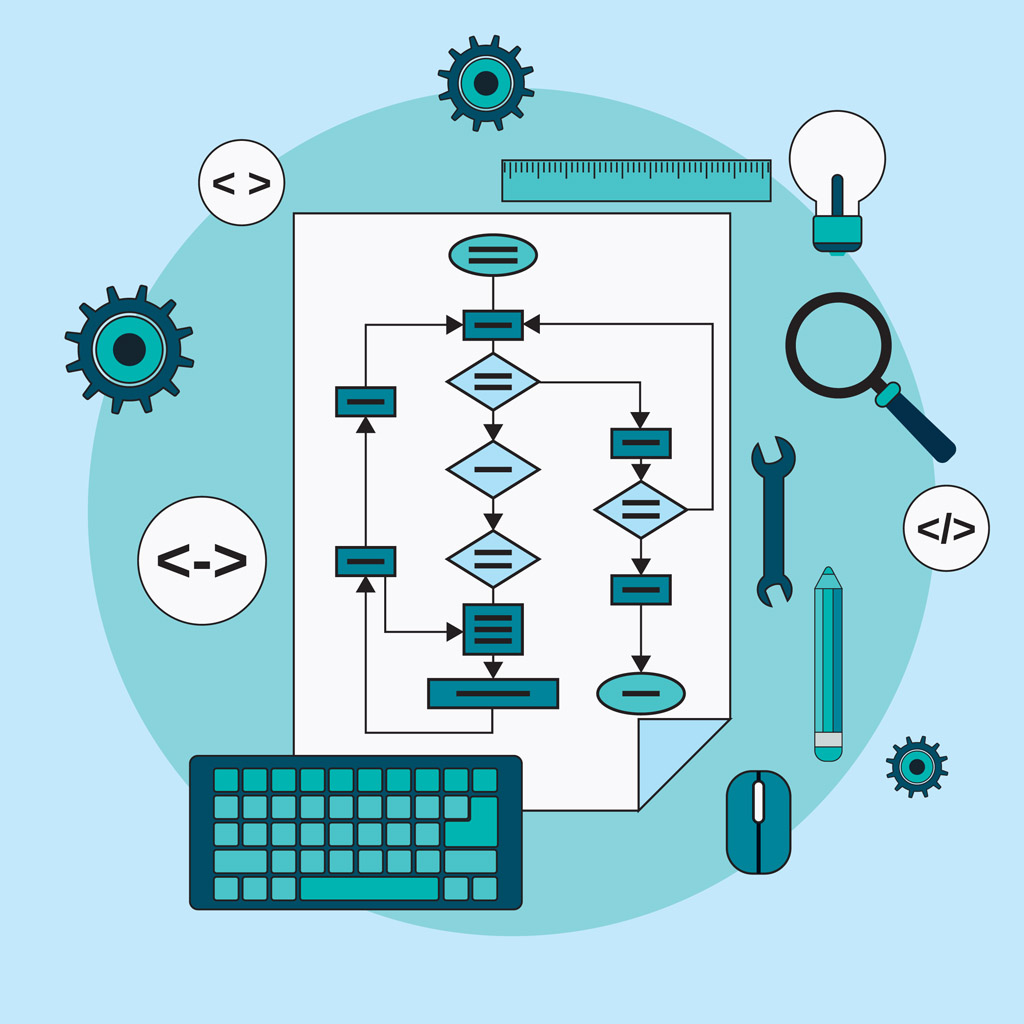
When you think about it, algorithmic logic surrounds us. For instance, anyone that has followed the steps of a recipe or used navigation software has used an algorithm.
As we know, computers are a major part of our lives, and are used to run many different types of objects in the real world, which we really take for granted. Algorithms are the basic building blocks of the computer programs that run these machines and devices. Some real-world examples of algorithms at work include:
Example
Autopilot algorithms
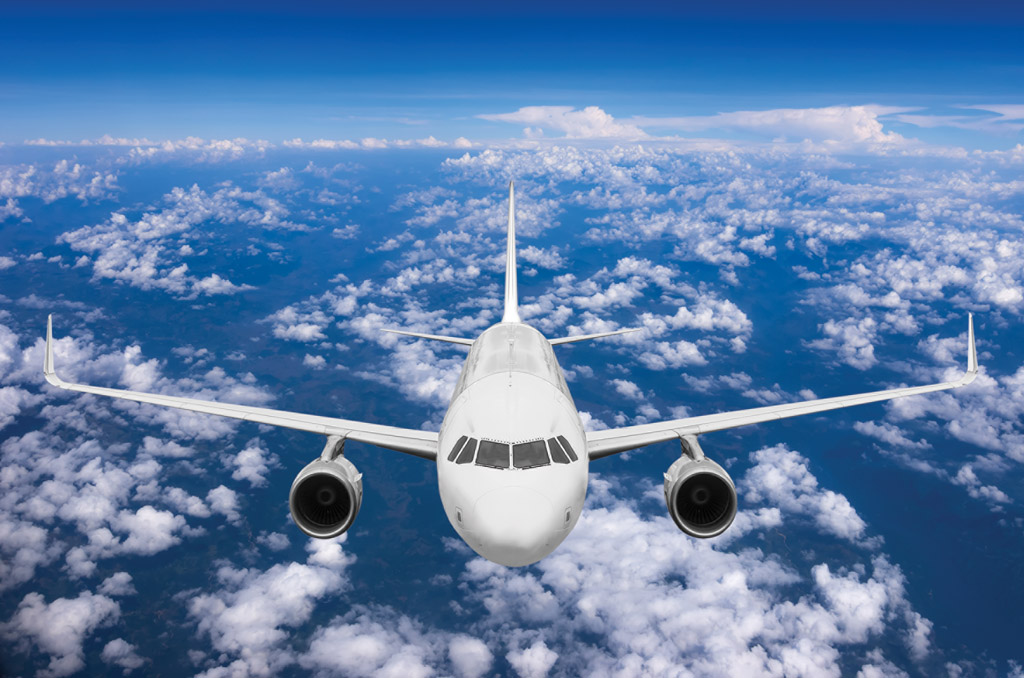
Most modern passenger aircraft today are fitted with autopilot systems that allow the aircraft to fly safely without direct input from the pilot. Such systems rely on computer programs to help implement an algorithm, which is designed to accept a range of inputs and deliver outputs, resulting in actions that steer the aircraft safely.
Inputs for such an algorithm might include the air speed, direction or tilt angle. The outputs might relate to engine thrust, flap angle or rudder changes. While looking at the wing on a passenger aircraft during flight you may have noticed the constant movement of a relatively small flap called an aileron. Under the control of an autopilot algorithm, this flap will continuously make changes to its angle, which adjusts the tilt of the aircraft. A constant feed of information into the algorithm will allow the program to update the output and change the aileron’s angle accordingly.
Without an efficient algorithm designed using mathematics and computer code, autopilot systems would not be the essential aeronautical tool that is in widespread use today.
Example
Driverless cars
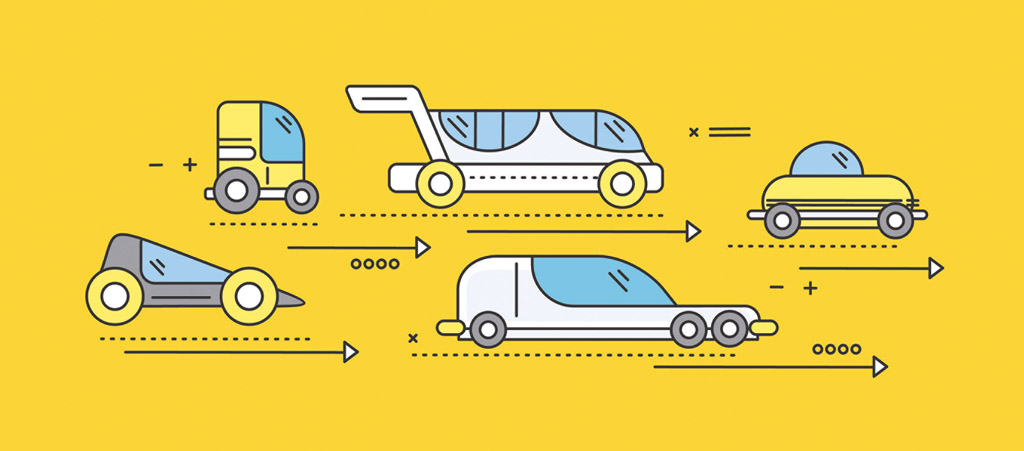
The vast majority of accidents in motor vehicles are due to driver error, which is one of the reasons why companies such as Google are experimenting with driverless cars. Such vehicles are currently being tested in a number of countries. The vehicles map their current position and use a range of sensors to determine the moving and stationary objects in the immediate area. This information is the input for computer-based algorithms that make predictions and test scenarios at a rapid rate. The efficiency of the computer code is critical in the running of the systems, which is why skilled programmers and mathematicians are responsible for their design. If the system detects a pedestrian, for example, the code needs to take into account the probability that this person could cross the road in front of the car. The software must choose the safest possible route at the safest speed.
Driverless cars have the potential to reduce road fatalities and increase the level of efficiency on our road networks. Parking would become less problematic, with a driverless car dropping you off at work and travelling to a remote parking spot somewhere else, before returning later in the day to pick you up.
Algorithms and programming exercise 2
Class discussion
Skills practised
- Understanding algorithms
- Applying algorithmic thinking
Discuss the two examples of algorithms used in important real-world applications. With so much at stake in both scenarios, can you predict the sorts of problems that would occur if the algorithms were not perfect?
Algorithms and programming exercise 3
Algorithms and flowcharts with a loop – convert to structured English
Skills practised
- Understanding algorithms
- Converting algorithms into structured English
A librarian wants to print out the current loans for a particular student. This will involve a search through all records of currently borrowed books. The algorithm below displays a loop as each book is checked for if it is borrowed by this student. It also includes a check if there are any more books to check – or an end point to the loop. Examine the algorithm and flowchart below. Fill in the missing text in the structured English box.
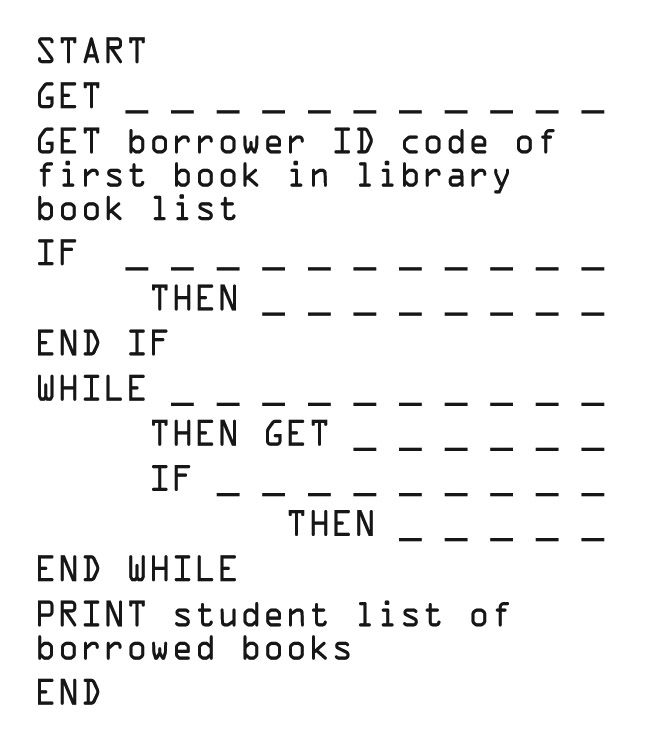
- The librarian keys in the student’s ID code.
- A search is made for the code in book records.
- A list of books borrowed by that student is printed.
- Enter student ID code.
- Check the first book in library book list to see if it is borrowed.
- If it is borrowed, is it borrowed by this student?
- If yes, add the book to the student list.
- If no, look at the next book.
- Continue while there are books to check.
- When finished checking all books, print the student list of borrowed books.
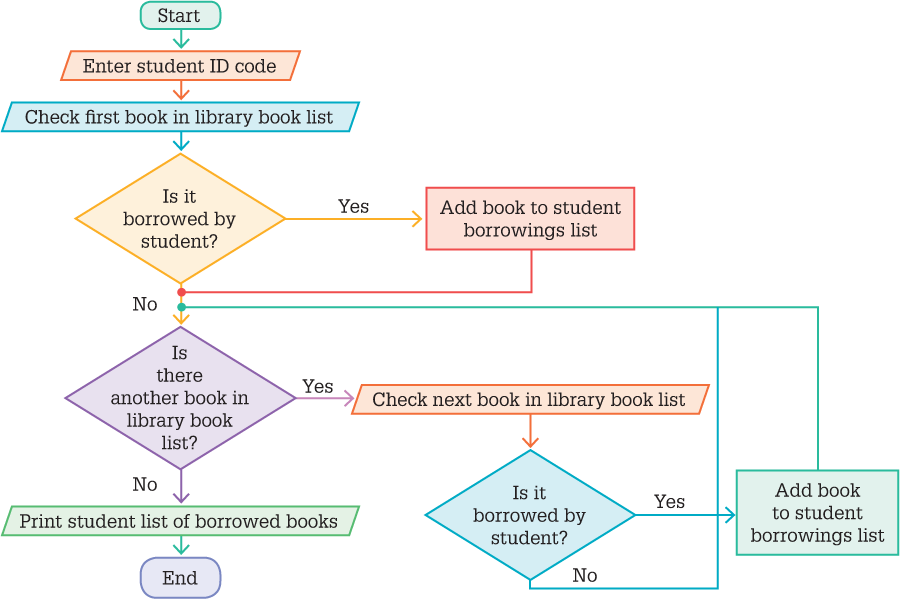
Flowchart symbols
Flowcharts for algorithms can get very complex. You may have already noticed the different shapes and when they are used.
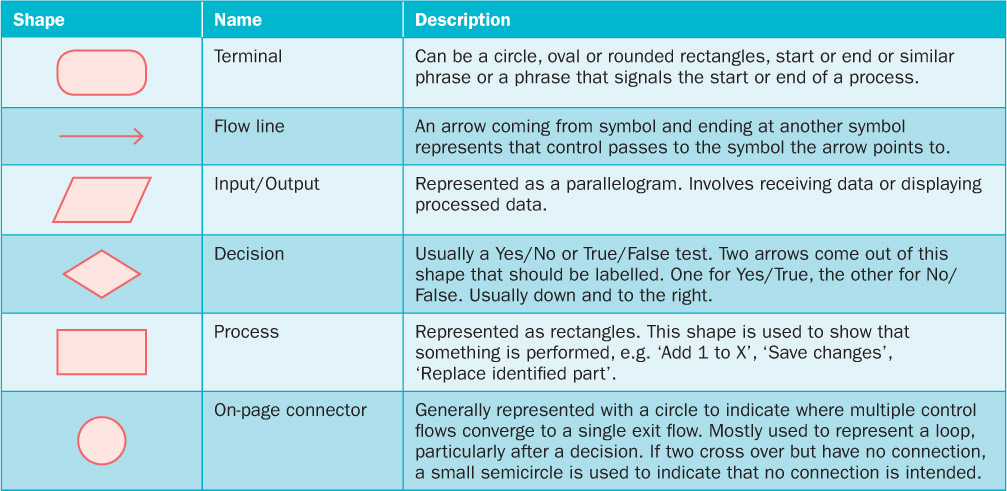
Shape | Name | Description |
---|---|---|
![]() |
Terminal | Can be a circle, oval or rounded rectangles, start or end or similar phrase or a phrase that signals the start or end of a process. |
![]() |
Flow line | An arrow coming from symbol and ending at another symbol represents that control passes to the symbol the arrow points to. |
![]() |
Input/Output | Represented as a parallelogram. Involves receiving data or displaying processed data. |
![]() |
Decision | Usually a Yes/No or True/False test. Two arrows come out of this shape that should be labelled. One for Yes/True, the other for No/False. Usually down and to the right. |
![]() |
Process | Represented as rectangles. This shape is used to show that something is performed, e.g. ‘Add 1 to X’, ‘Save changes’, ‘Replace identified part’. |
![]() |
On-page connector | Generally represented with a circle to indicate where multiple control flows converge to a single exit flow. Mostly used to represent a loop, particularly after a decision. If two cross over but have no connection, a small semicircle is used to indicate that no connection is intended. |
Tip: You can easily draw flowcharts using shape tools in Microsoft Word or Google Drawings. Refer back to Book 1, Module 4: Drawing tools, Exercises 1–2 if you need a refresher.
Algorithms and programming exercise 4
Algorithms, flowcharts and structured English
Skills practised
- Creating algorithms
- Drawing flowcharts
- Converting flowcharts into structured English
- Testing algorithms
Write an algorithm and draw a corresponding flowchart that works for the following procedure. Convert your flowchart into structured English. Remember to test your results and work through them to make sure they work. Use various scenarios. For example, suppose for the example in Exercise 3 that the library only has one book – strange but possible. Suppose the student had no books borrowed – what will happen then? Should some steps have been added to the flowchart or algorithm?
Algorithms and programming exercise 5
Algorithms, flowcharts and structured English
Skills practised
- Creating algorithms
- Drawing flowcharts
- Converting flowcharts into structured English
- Testing algorithms
Write an algorithm and draw a corresponding flowchart that works for each of the following procedures. Convert your flowcharts into structured English. Remember to test your results and work through them to make sure they work.
A salesperson is checking the expiry date of a credit card to be used to pay for a purchase.
- The salesperson enters the date of expiry of the credit card.
- The expiry date on the card is compared to today’s date.
- The result is displayed on the cash register screen.
Algorithms and programming exercise 6
Algorithms, flowcharts and structured English
Skills practised
- Creating algorithms
- Drawing flowcharts
- Converting flowcharts into structured English
- Testing algorithms
Write an algorithm and draw a corresponding flowchart that works for the following procedure. Convert your flowchart into structured English. Remember to test your results and work through them to make sure they work. Use various scenarios.
A fee for internet data usage is to be calculated and charged to the customer. So the amount of gigabytes used by the customer needs to be accessed. If less than 8 GB are used then there is no extra charge. If between 8 and 25 GB are used, the charge is an extra $2 per GB. If more than 25 GB are used then the charge is $5 per GB.
Once the fee is determined, the charge is added to the customer’s account for the month.